The error “Client network socket disconnected before secure TLS connection was established” indicates something went wrong when setting up a secure connection with the server you’re communicating with. This can happen because of an unstable internet connection, wrong security settings on the server, or things like firewalls getting in the way.
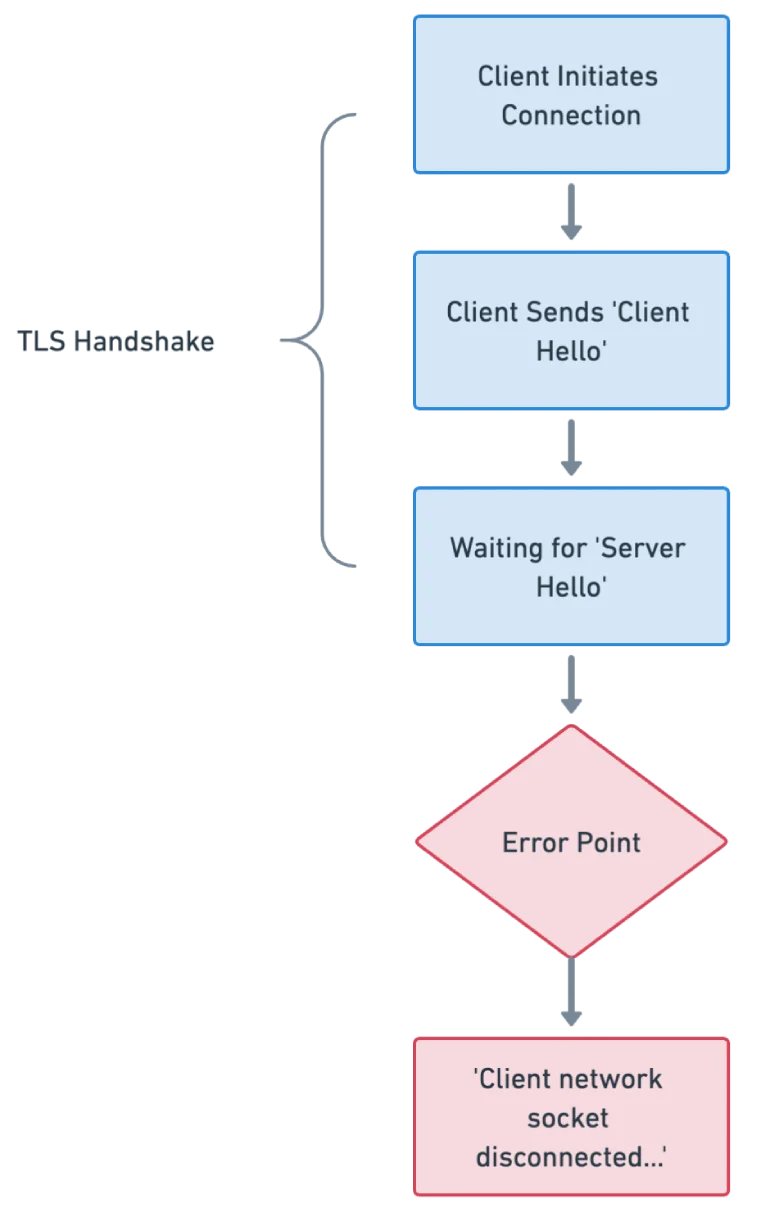
Although this blog post will use Node.js in examples to discuss this error, the underlying cause is related to the network layer rather than a language-specific issue. You can get this error in any programming language that supports network operations and TLS (Transport Layer Security) for secure connections.
What causes “Client network socket disconnected…”?
Here are some of the typical causes:
- Timeout Issues: The client took too long to establish a connection with the server. This could be due to network latency, server overload, or other network-related issues.
- Proxy or VPN Interference: If the client is behind a proxy or using a VPN, these can sometimes interfere with or disrupt the TLS handshake process.
- Firewall or Security Software: Firewalls or security software on the client or server side may block the connection or interrupt the TLS handshake.
- SSL Certificate Problems: Issues with the server's SSL certificate, such as it being expired, self-signed, or not properly configured, can prevent a successful TLS connection.
- Incompatible TLS Versions: If the Node.js client and the server are using incompatible TLS versions or cipher suites, the handshake will fail.
- Server Configuration Issues: Misconfigurations on the server-side, especially related to TLS/SSL settings, can cause this error.
- Incorrect Client Configuration: In Node.js, if the client (for example, using the
https
oraxios
module) is not correctly configured for the specific requirements of the server (like custom headers, or specific SSL/TLS settings), the connection can fail.
How to fix “Client network socket disconnected…”
First, let’s get the easy stuff out of the way:
- Check the network connectivity and configuration on both the client and server sides
- Verify that there are no firewalls or security software blocking the connection
If there’s nothing wrong with your internet, you’ll need to look into more technical aspects.
3. Inspect Server SSL/TLS Configuration
Make sure the server is correctly configured for SSL/TLS. Check that it is listening on the correct port (usually 443 for HTTPS) and that its SSL certificate is valid, not expired, and trusted by a known certificate authority. You can use online SSL verification tools like https://www.ssllabs.com/ssltest/ to check the server's certificate and configuration.
4. Check TLS Version Compatibility
When your Node.js client application is trying to connect to an external server e.g. a web API, a third-party service, a database server, etc., ensure that the TLS version and cipher suites supported by your Node.js application are compatible with those supported by the server.
Sometimes, older servers might use outdated TLS versions that are not supported by modern clients. Once you know the TL version, you can specify the TLS version in your Node.js code by creating a custom HTTPS agent with specific TLS settings. Here’s how to do it using https
:
const https = require('https');
// Create a custom HTTPS agent with specific TLS settings
const agent = new https.Agent({
secureProtocol: 'TLSv1_2_method' // Specify the TLS version, e.g., 'TLSv1_2_method' for TLS 1.2
});
// Making a request with the custom agent
https.get('https://example.com', { agent }, (res) => {
console.log('StatusCode:', res.statusCode);
// Handle response...
}).on('error', (e) => {
console.error(e);
});
And here’s how to do it using axios
:
const axios = require('axios');
const https = require('https');
// Create a custom HTTPS agent with specific TLS settings
const httpsAgent = new https.Agent({
secureProtocol: 'TLSv1_2_method' // Specify the TLS version, e.g., 'TLSv1_2_method' for TLS 1.2
});
// Making an axios request with the custom agent
axios.get('https://example.com', { httpsAgent })
.then(response => {
console.log('Response data:', response.data);
})
.catch(error => {
console.error('Error:', error);
});
In these examples, TLSv1_2_method
specifies TLS version 1.2. You can adjust this to the desired TLS version like TLSv1_1_method
, TLSv1_2_method
, or TLSv1_3_method
.
5. Analyze server logs
If you have access to the server, check the logs on the server side for any indications of why the connection might be failing. Server logs can provide insights into issues like failed handshakes, unsupported protocols, or cipher suite mismatches.
6. Implement retry mechanisms
Network requests might fail due to temporary problems like brief network disconnections or server overload. Here's an example of how you could implement a simple retry mechanism for an HTTP request using Axios:
const axios = require('axios');
const MAX_RETRIES = 3; // Maximum number of retries
// Function to make a request with retry logic
async function makeRequestWithRetry(url, retries = MAX_RETRIES) {
try {
const response = await axios.get(url);
console.log('Response:', response.data);
return response.data;
} catch (error) {
if (retries > 0) {
console.log(Retrying... attempts left: ${retries - 1}
);
await new Promise(resolve => setTimeout(resolve, 1000)); // Wait for 1 second before retrying
return makeRequestWithRetry(url, retries - 1);
} else {
throw new Error('Max retries reached. ' + error.message);
}
}
}
// Usage example
makeRequestWithRetry('https://example.com')
.then(data => console.log('Data received:', data))
.catch(error => console.error('Failed:', error.message));
As a last resort, set rejectUnauthorized to false
If you tried all the above and you still can’t resolve the issue, setting rejectUnauthorized
to false
allows Node.js to accept and establish connections with servers that have invalid or self-signed SSL certificates, effectively bypassing SSL/TLS certificate validation.
Doing this compromises security and should be avoided, especially in production environments, but if you just want to test or in some cases, particularly within controlled and secure internal networks (like an internal company network), where you are sure about the integrity and safety of the servers you're connecting to, this setting might be used.
Here’s how you can do this using axios
:
const axios = require('axios');
const https = require('https');
// Create a custom HTTPS agent with rejectUnauthorized set to false
const httpsAgent = new https.Agent({
rejectUnauthorized: false // Bypasses SSL certificate validation
});
// Making an Axios request with the custom agent
axios.get('https://example.com', { httpsAgent })
.then(response => {
console.log('Response:', response.data);
})
.catch(error => {
console.error('Error:', error);
});
Again, do this with extreme caution. Bypassing SSL certificate validation introduces significant security risks, especially in production environments. It makes your application vulnerable to man-in-the-middle attacks. It’s always better to use valid, trusted SSL certificates and to fix any certificate-related issues rather than bypassing security checks.
Track, Analyze and Manage Errors With Rollbar
Managing errors and exceptions in your code is challenging. It can make deploying production code an unnerving experience. Being able to track, analyze, and manage errors in real-time can help you to proceed with more confidence. Rollbar automates error monitoring and triaging, making fixing network errors easier than ever. Try it today!