Introduction to Java Compile-time Errors
Over the past two and a half decades, Java has consistently been ranked as one of the top 3 most popular programming languages in the world [1], [2]. As a compiled language, any source code written in Java needs to be translated (i.e., compiled) into machine code before it can be executed. Unlike other compiled languages where programs are compiled directly into machine code, the Java compiler converts the source code into intermediate code, or bytecode, which is then translated into machine code for a specific platform by the Java Virtual Machine (JVM). This, in the simplest of terms, is how Java achieves its platform independence (Fig. 1).
One advantage that comes with being a compiled language is the fact that many errors stemming from incorrect language syntax and semantics (such as “illegal start of expression”) can be captured in the compilation process, before a program is run and they inadvertently find their way into production environments. Since they occur at the time of compilation, these errors are commonly referred to as compile-time errors.
The Java compiler can detect syntax and static semantic errors, although it is incapable of recognizing dynamic semantic errors. The latter are logical errors that don’t violate any formal rules and as such cannot be detected at compile-time; they only become visible at runtime and can be captured by well-designed tests.
When it encounters an error it can recognize, the Java compiler generates a message indicating the type of error and the position in the source file where this error occurred. Syntax errors are the easiest to detect and correct.
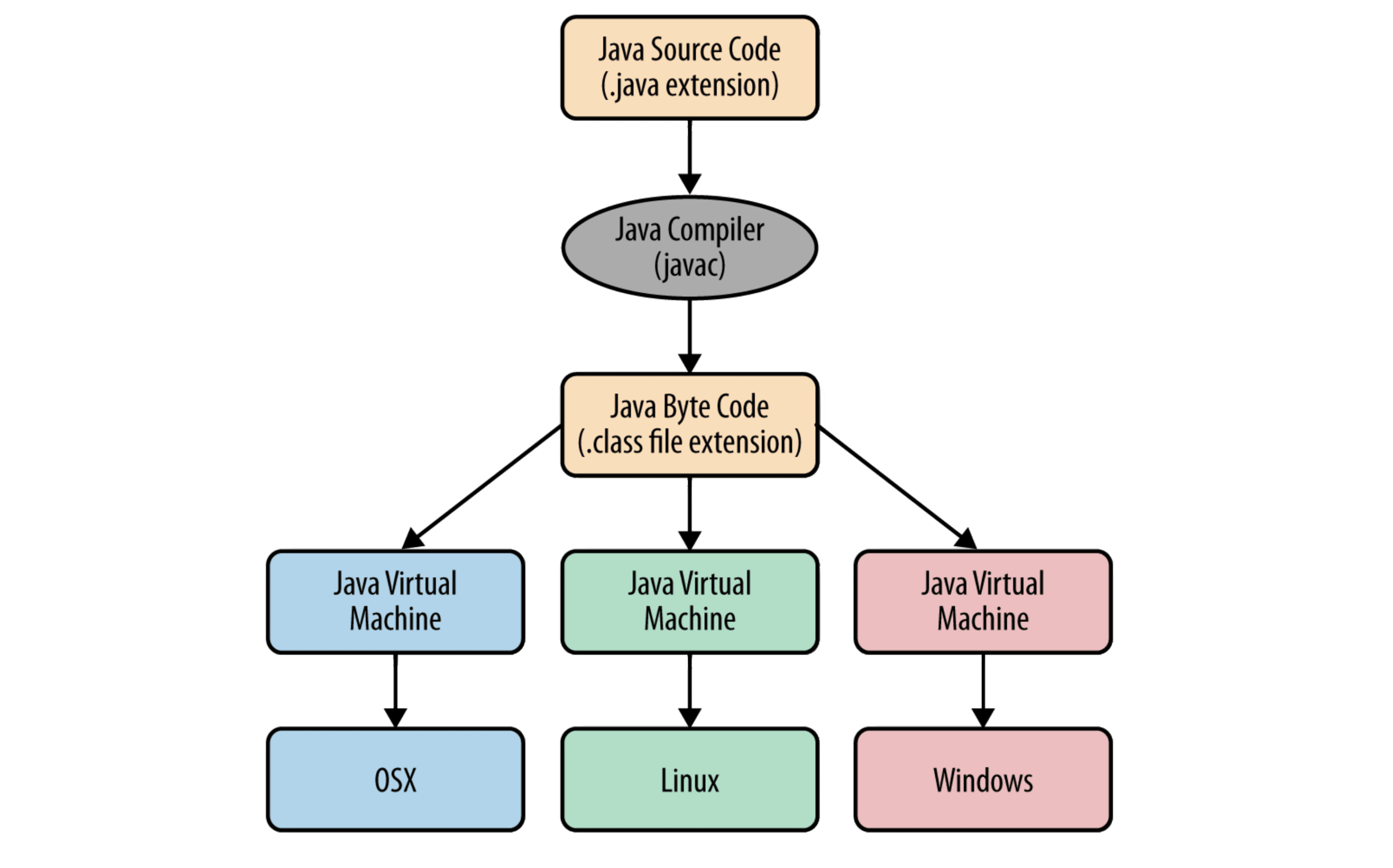
 
Illegal Start of Expression: What is it?
Expressions are one of the main building blocks of any Java application. These are constructs that compute values and control the execution flow of the program. As its name implies, the “illegal start of expression” error refers to an expression that violates some rule at the point where it starts, usually right after another expression ends; the assumption here is that the preceding expression is correct, i.e., free of errors.
This error is analogous to starting an English sentence with an invalid word, like saying "Went the dog the park". Just as a sentence must begin with a valid structure and follow certain rules to be understood, a Java statement must also begin with the right structure and rules to be executed correctly.
What Causes Illegal Start of Expression
The “illegal start of expression” error often arises from an insufficient familiarity with the language or due to basic negligence. The cause for this error can usually be found at the beginning of an expression or, in some cases, the entire expression might be incorrect or misplaced.
Some common scenarios where this error can occur are:
- Invalid usage of access modifiers. For example, using access modifiers on local variables.
- Incorrect nesting. For example, using nested methods throws the error since Java doesn’t support them.
- Incorrect block structure. For example, using mismatched braces.
- Invalid variable declarations or assignments. For example, initializing a string without quotes.
Illegal Start of Expression Examples
Example One: Access modifiers on local variables
A local variable in Java is any variable declared inside the body of a method or, more generally, inside a block. A local variable’s accessibility is predetermined by the block in which it is declared—the variable can be accessed strictly within the scope of its enclosing block. Therefore, access modifiers have no use here and, if introduced, will raise the “illegal start of expression” error (Fig. 2(a)). Removing the access modifier (as shown on line 5 in Fig. 2(b)) resolves the Java error.
(a)
package rollbar;
public class AccessModifierOnLocalVariable {
public static void main(String... args) {
private String localString = "MyString";
System.out.println(localString);
}
}
AccessModifierOnLocalVariables.java:5: error: illegal start of expression
private String localString = "MyString";
^
(b)
package rollbar;
public class AccessModifierOnLocalVariable {
public static void main(String... args) {
String localString = "MyString";
System.out.println(localString);
}
}
Output: MyString
 
Example Two: Nested methods
Unlike some other languages (most notably functional languages), Java does not allow direct nesting of methods, as shown in Fig. 3(a). This violates Java’s scoping rules and object-oriented approach.
There are two main ways of addressing this issue. One is to move the inner method to an appropriate place outside the outer method (Fig. 3(b)). Another one is to replace the inner method with a lambda expression assigned to a functional interface (Fig. 3(c)).
(a)
package rollbar;
public class MethodInsideAnotherMethod {
public static void main(String... args) {
static double root(int x) {
return Math.sqrt(x);
}
System.out.println(root(9));
}
}
MethodInsideAnotherMethod.java:5: error: illegal start of expression
static double root(int x) {
^
(b)
package rollbar;
public class MethodInsideAnotherMethod {
public static void main(String... args) {
System.out.println(root(9));
}
static double root(int x) {
return Math.sqrt(x);
}
}
Output: 3.0
(c)
package rollbar;
import java.util.function.Function;
public class MethodInsideAnotherMethod {
public static void main(String... args) {
Function<Integer, Double> root = x -> Math.sqrt(x);
System.out.println(root.apply(9));
}
}
Output: 3.0
 
Example Three: Missing braces
According to Java syntax, every block has to start and end with an opening and a closing curly brace, respectively. If a brace is omitted, the compiler won’t be able to identify the start and/or the end of a block, which will result in an illegal start of expression
error (Fig. 4(a)). Adding the missing brace fixes the error (Fig. 4(b)).
(a)
package rollbar;
public class MissingCurlyBrace {
static int fibonacci(int n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
public static void main(String... args) {
System.out.println(fibonacci(10));
}
}
MissingCurlyBrace.java:10: error: illegal start of expression
public static void main(String... args) {
^
(b)
package rollbar;
public class MissingCurlyBrace {
static int fibonacci(int n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
public static void main(String... args) {
System.out.println(fibonacci(10));
}
}
Output: 55
 
Example Four: Array creation
Traditionally, array creation in Java is done in multiple steps, where the array data-type and size are declared upfront and its values initialized afterwards, by accessing its indices. However, Java allows doing all of these operations at once with a succinct, albeit somewhat irregular-looking, syntax (Fig. 5(a)).
While very convenient, this syntactical idiosyncrasy only works as a complete inline expression and will raise the illegal start of expression
error if used otherwise (Fig. 5(b)). This syntax cannot be used to initialize values of an array whose size has already been defined, because one of the things it tries to do is exactly that—assign a size to the array.
The only other scenario in which this syntax may be used is to overwrite an existing array with a new one, by prefixing it with the new
directive (Fig. 5(c)).
(a)
package rollbar;
import java.util.Arrays;
public class ArrayInitialization {
public static void main(String[] args) {
int[] integers = {1, 2, 3, 4, 5}; //Declaring and initializing array at once
System.out.println(Arrays.toString(integers));
}
}
Output: [1, 2, 3, 4, 5]
(b)
package rollbar;
import java.util.Arrays;
public class ArrayInitialization {
public static void main(String... args) {
int[] integers = new int[5];
integers = {1, 2, 3, 4, 5}; //Trying to initialize array with size defined previously
System.out.println(Arrays.toString(integers));
}
}
ArrayInitialization.java:8: error: illegal start of expression
integers = {1, 2, 3, 4, 5};
^
(c)
package rollbar;
import java.util.Arrays;
public class ArrayInitialization {
public static void main(String... args) {
int[] integers = new int[5];
integers = new int[]{1,2,3,4,5}; //Overwriting existing array using new directive
System.out.println(Arrays.toString(integers));
}
}
Output: [1, 2, 3, 4, 5]
 
How to Fix Illegal Start of Expression
The following steps should be followed to resolve the “illegal start of expression” error:
- Check the stack trace. Inspect the error stack trace to detect where the issue exists in code.
- Verify syntax and structure. Check the lines in code throwing the error to address the issues.
- Check usage of access modifiers. Ensure that access modifiers are used correctly and are not used inside methods.
- Inspect nesting structure. When working with nested blocks of code, ensure the structure is correct. Remember that Java does not support nested methods.
- Verify block structure. Make sure that code blocks are structured correctly e.g. ensure that starting and closing braces are matching properly.
- Examine variable declarations and assignments. Address any invalid variable declarations or assignments.
Summary
Being a compiled language, Java has an advantage over other languages in its ability to detect and prevent certain errors from slipping through into production. One such error is the “illegal start of expression” error which belongs to the category of syntax errors detected at compile time. Common examples have been presented in this article along with explanations of their cause and ways to resolve them.
 
Track, Analyze and Manage Errors With Rollbar
Managing errors and exceptions in your code is challenging. It can make deploying production code an unnerving experience. Being able to track, analyze, and manage errors in real-time can help you to proceed with more confidence. Rollbar automates error monitoring and triaging, making fixing Java errors easier than ever. Sign Up Today!
 
References
[1] TIOBE Software BV, “TIOBE Index for October 2021: TIOBE Programming Community index,” TIOBE Software BV. [Online]. Available: https://www.tiobe.com/tiobe-index/. [Accessed Oct. 28, 2021].
[2] Statistics & Data, “The Most Popular Programming Languages – 1965/2021,” Statistics and Data. [Online]. Available: https://statisticsanddata.org/data/the-most-popular-programming-languages-1965-2021/. [Accessed Oct. 28, 2021].
[3] C. Saternos, Client-Server Web Apps with JavaScript and Java. Sebastopol, CA: O’Reilly Media, Inc., 2014, Ch. 4, p.59