Introduction to Method and Constructor Signatures
In computer programming, a function is a set of instructions that can be invoked to perform a particular task. In object-oriented programming (OOP), a method is a function that is typically associated with an object and models its behavior [1]. In Java, methods can also be static, in which case they are part of a class definition and do not require an object to be created before they are invoked.
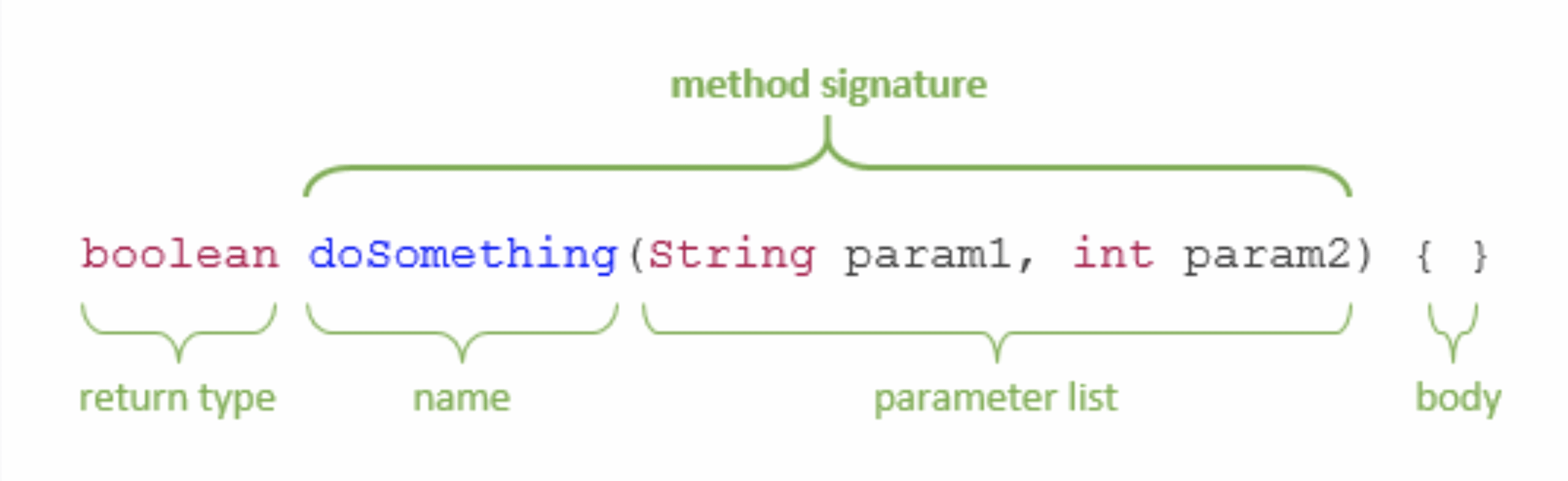
 
Every method has a unique method signature, which consists of the method name and its input parameters, which can be zero or more. Java methods also have return types, but these are not considered a part of the method signature, as it would be impossible for a compiler to distinguish between overloaded methods in certain contexts [2]. Below is an example of a method declaration with a method signature consisting of the method name “doSomething” and two method parameters; the 1st one of type String
and name “param1,” and the 2nd one of type int
with the name “param2.” It is important to note that method parameters always have a type and a name, and the order in which they appear in the method declaration matters.
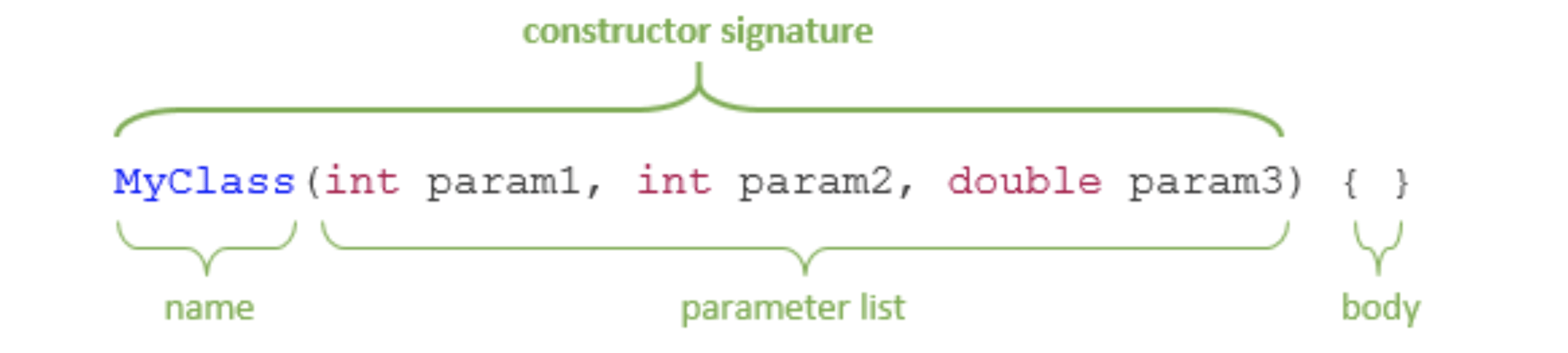
 
Constructors are a special type of method invoked to create objects from a class. Constructors have no return type and always use the name of the class in which they are declared [3]. Similarly to method signatures, a constructor signature is made up of the constructor name and a comma-delimited list of input parameters enclosed in parentheses.
 
Method X in Class Y Cannot be Applied to Given Types & Constructor X in Class Y Cannot be Applied to Given Types
When performing semantic analysis, the Java compiler checks all method invocations for correctness. Whenever a method invocation doesn’t match the corresponding method signature, the method X in class Y cannot be applied to given types
error is raised. This error is triggered by a method call attempted with the wrong number, type, and/or order of arguments.
Arguments are the actual data values passed to the method’s parameters during invocation. These have to match (or be safely convertible to) the parameters in the method signature, otherwise the aforementioned error will be raised.
Similarly, the constructor X in class Y cannot be applied to given types
error is triggered whenever a constructor invocation doesn’t match the corresponding constructor signature, and the arguments passed on to the constructor don’t match the predefined parameters.
The messages produced upon compilation for these errors provide helpful information by specifying the following fields:
- “required”—the formal parameters expected by the method;
- “found”—the actual parameters (arguments) used to call the method; and
- “reason”—the specific cause for compilation failure.
 
Examples
Method X in Class Y Cannot be Applied to Given Types
The signature of the method on line 9 in Fig. 1 denotes a single String
parameter, which means a single argument of type String
is expected. If this method is invoked without an argument, the method X in class Y cannot be applied to given types
error is raised, with an appropriate message that reads “actual and formal argument lists differ in length (Fig. 1(a)).” Likewise, if the greetPerson
method is invoked with more than one argument, the same error will be raised (Fig. 2(b)). And if an argument of a different type is used instead, the same type of error is raised and the reason states an “argument mismatch” between the expected (required) and actual (found) argument, as shown in Fig. 1(c). Intuitively, calling the method with the appropriate number and type of arguments—a single String
value in this particular case—rectifies the issue (Fig. 1(d)).
(a)
1
2
3
4
5
6
7
8
9
10
11
package rollbar;
public class MismatchedMethodSig {
public static void main(String... args) {
greetPerson();
}
static void greetPerson(String name) {
System.out.println("Hello " + name);
}
}
MismatchedMethodSig.java:6: error: method greetPerson in class MismatchedMethodSig cannot be applied to given types;
greetPerson();
^
required: String
found: no arguments
reason: actual and formal argument lists differ in length
(b)
1
2
3
4
5
6
7
8
9
10
public class MismatchedMethodSig {
public static void main(String... args) {
greetPerson("John", 123);
}
static void greetPerson(String name) {
System.out.println("Hello " + name);
}
}
MismatchedMethodSig.java:6: error: method greetPerson in class MismatchedMethodSig cannot be applied to given types;
greetPerson("John", 123);
^
required: String
found: String,int
reason: actual and formal argument lists differ in length
(c)
1
2
3
4
5
6
7
8
9
10
11
12
package rollbar;
public class MismatchedMethodSig {
public static void main(String... args) {
greetPerson(123);
}
static void greetPerson(String name) {
System.out.println("Hello " + name);
}
}
MismatchedMethodSig.java:6: error: method greetPerson in class MismatchedMethodSig cannot be applied to given types;
greetPerson(123);
^
required: String
found: int
reason: argument mismatch; int cannot be converted to String
(d)
1
2
3
4
5
6
7
8
9
10
11
12
package rollbar;
public class MismatchedMethodSig {
public static void main(String... args) {
greetPerson("John");
}
static void greetPerson(String name) {
System.out.println("Hello " + name);
}
}
Hello John
 
Constructor X in Class Y Cannot be Applied to Given Types
Just as with ordinary methods, constructors need to be invoked with the correct number, type, and order of arguments. Fig. 2.1 shows how calling a constructor by omitting an argument triggers the constructor X in class Y cannot be applied to given types
error.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
package rollbar;
public class MismatchedConstructorSig {
public static void main(String... args) {
Person jill = new Person("Jill"); // omitting the "age" argument
System.out.println(jill);
}
static class Person {
private String name;
private int age;
Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return String.format("Person { name='%s', age=%d }", name, age);
}
}
}
MismatchedConstructorSig.java:6: error: constructor Person in class Perso
n cannot be applied to given types;
Person jill = new Person("Jill"); // omitting the "age" argument
^
required: String,int
found: String
reason: actual and formal argument lists differ in length
The example in Fig. 2.2 demonstrates how passing an argument of a data type different than what the method expects results in the same error. The “reason” in the error message here reads “argument mismatch; possible lossy conversion from double to int” which means that the decimal value 25.5 cannot be converted to an integer without losing some of the information, which makes sense. However, Fig 2.5 shows how the opposite works, i.e., how an integer can safely be converted to a double decimal value, and the compiler doesn’t see this as an error.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
package rollbar;
public class MismatchedConstructorSig {
public static void main(String... args) {
Person jill = new Person("Jill", 25.5); // expected int
System.out.println(jill);
}
static class Person {
private String name;
private int age;
Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return String.format("Person { name='%s', age=%d }", name, age);
}
}
}
MismatchedConstructorSig.java:6: error: constructor Person in class Perso
n cannot be applied to given types;
Person jill = new Person("Jill", 25.5); // expected int
^
required: String,int
found: String,double
reason: argument mismatch; possible lossy conversion from double to int
Lastly, mixing up the order of arguments can often result in the constructor X in class Y cannot be applied to given types
error, as can be seen in Fig 2.3. This is a dangerous mistake, as in certain scenarios, namely those where all arguments are of the same type, no compilation error will be raised and consequences will emerge at run time.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
package rollbar;
public class MismatchedConstructorSig {
public static void main(String... args) {
Person jill = new Person(25, "Jill");
System.out.println(jill);
}
static class Person {
private String name;
private int age;
Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return String.format("Person { name='%s', age=%d }", name, age);
}
}
}
MismatchedConstructorSig.java:6: error: constructor Person in class Perso
n cannot be applied to given types;
Person jill = new Person(25, "Jill");
^
required: String,int
found: int,String
reason: argument mismatch; int cannot be converted to String
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
package rollbar;
public class MismatchedConstructorSig {
public static void main(String... args) {
Person jill = new Person("Jill", 25);
System.out.println(jill);
}
static class Person {
private String name;
private int age;
Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return String.format("Person { name='%s', age=%d }", name, age);
}
}
}
Person { name='Jill', age=25 }
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
package rollbar;
public class MismatchedConstructorSig {
public static void main(String... args) {
Person jill = new Person("Jill", 25); // expected double
System.out.println(jill);
}
static class Person {
private String name;
private double age;
Person(String name, double age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return String.format("Person { name='%s', age=%s }", name, age);
}
}
}
Person { name='Jill', age=25.0 }
 
Summary
Methods and constructors play a crucial role in creating and manipulating objects in Java. Every method and constructor is uniquely identified by its signature, which is checked against all method and constructor invocations at compilation time. Whenever there is a mismatch between the formal parameters in a signature and the actual parameters (arguments) in an invocation of the corresponding method or constructor, the method X in class Y cannot be applied to given types
or the constructor X in class Y cannot be applied to given types
error are introduced, respectively. The accompanying error messages contain clear and concise information which helps resolve the issue, by fixing the method or constructor call in question.
 
Track, Analyze and Manage Errors With Rollbar
Managing errors and exceptions in your code is challenging. It can make deploying production code an unnerving experience. Being able to track, analyze, and manage errors in real-time can help you to proceed with more confidence. Rollbar automates error monitoring and triaging, making fixing Java errors easier than ever. Sign Up Today!
 
References
[1] Oracle, 2021. Lesson: Object-Oriented Programming Concepts (The Java™ Tutorials > Learning the Java Language), Oracle and/or its affiliates. [Online]. Available: https://docs.oracle.com/javase/tutorial/java/concepts/. [Accessed: Nov. 29, 2021]
[2] Oracle, 2021. Defining Methods (The Java™ Tutorials > Learning the Java Language > Classes and Objects), Oracle and/or its affiliates. [Online]. Available: https://docs.oracle.com/javase/tutorial/java/javaOO/methods.html. [Accessed: Nov. 29, 2021]
[3] Oracle, 2021. Providing Constructors for Your Classes (The Java™ Tutorials > Learning the Java Language > Classes and Objects), Oracle and/or its affiliates. [Online]. Available: https://docs.oracle.com/javase/tutorial/java/javaOO/constructors.html. [Accessed: Nov. 29, 2021]