An exception is an unwanted or unexpected event that occurs during the execution of a program which changes its normal flow. Exception handling is the mechanism to respond to and investigate the occurrence and cause of an exception.
Generally, exceptions are of two types - checked and unchecked. Scala only allows unchecked exceptions - this means that there is no way to know if a method throws an unhandled exception at compile-time
It is best practice in Scala to handle exceptions using a try{...} catch{...}
block, similar to how it is used in Java, except that the catch
block uses pattern matching to identify and handle exceptions.
 
Scala Exceptions Hierarchy
All exception and error types in Scala are subclasses of the Throwable
class, the base class of the Scala exception hierarchy:
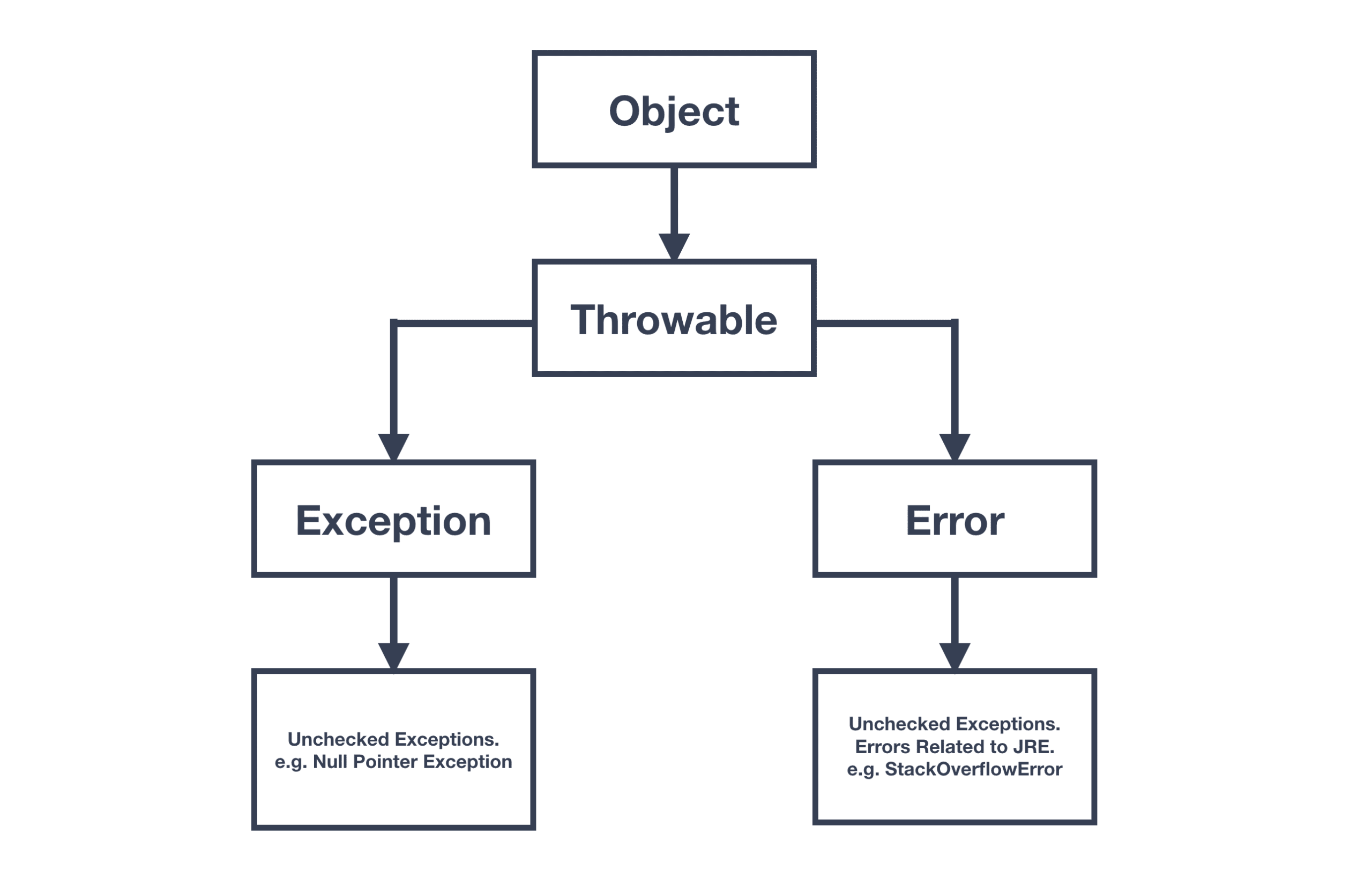
As evident from the diagram shown above, one branch of the hierarchy under Throwable
is headed by Exception
, which is the class used for exceptional conditions that programs should catch. An example of an exception is NullPointerException
.
Another branch is Error
, which is used by the Java Virtual Machine (JVM) to indicate errors that are related to the Java runtime environment itself (JRE). An example of an error is StackOverflowError
.
 
Throwing Exceptions in Scala
Throwing exceptions in Scala is very similar to how it's done in Java. An exception object is created and thrown using the throw
keyword. Here is an example:
throw new IllegalArgumentException
 
Scala try/catch Construct
Scala allows handling exceptions using a single try/catch block. Exceptions can then be pattern matched using case blocks instead of providing a separate catch
clause for each exception. Here's an example:
object ScalaExceptionExample {
def main(args: Array[String]) {
try {
val file = new FileReader("myfile.txt")
} catch {
case e: FileNotFoundException => println("FileNotFoundException occurred")
case e: IOException => println("IOException occurred")
}
}
}
Running the above code leads to the following output:
FileNotFoundException occurred
As it can be seen from the example, the try/catch block works similar to other languages. The body in the try
block is executed and if it throws an exception, catch
clauses are executed.
 
Scala finally Clause
An expression can be wrapped with the finally
clause if some part of the code needs to be executed irrespective of what happens before and how the expression terminates. This can especially be useful to close resources like database connections. Here's an example:
object ScalaExceptionExample {
def main(args: Array[String]) {
try {
val file = new FileReader("myfile.txt")
} catch {
case e: FileNotFoundException => println("FileNotFoundException occurred")
case e: IOException => println("IOException occurred")
} finally {
println("In finally block")
}
}
}
Running the above code leads to the following output:
FileNotFoundException occurred
In finally block
 
Scala Custom Exceptions
Scala allows developers to create their own custom exceptions. To declare a custom exception, the Exception
class needs to be extended. In the custom class, the conditions to throw the exception and a message can be defined. Here's an example:
class MyCustomException(s: String) extends Exception(s) {}
class MyCustomExceptionExample {
@throws(classOf[MyCustomException])
def validate(age: Int) {
if (age < 0) {
throw new MyCustomException("Age must be positive")
}
}
}
object MyObject {
def main(args: Array[String]) {
var mcee = new MyCustomExceptionExample()
try {
mcee.validate(-1)
} catch {
case e: Exception => println("Exception occurred : " + e)
}
}
}
Running the above code leads to the following output:
Exception occurred : MyCustomException: Age must be positive
 
Track, Analyze and Manage Errors With Rollbar
Managing errors and exceptions in your code is challenging. It can make deploying production code an unnerving experience. Being able to track, analyze, and manage errors in real-time can help you to proceed with more confidence. Rollbar automates Scala error monitoring and triaging, making fixing Scala exceptions easier than ever.