In the early days of the web, the idea of performance was relatively straightforward. Pages were static, and the most dynamic thing you might encounter was a blinking banner ad. But as the web evolved, so did our ambitions. Today it's not just about building web pages anymore; it's about crafting experiences. Load speed time and search engine optimization (SEO) matter just as much as the content on the page.
Thus, the choice between React and Next.js is an important one, with real-world implications. Let's explore how the rendering techniques of these two frameworks impact performance, user experience, and SEO and crown a winner.
Next.js vs. React Rendering Comparison
When a user goes to your website, their browser has to wait for your server to send a bundle of code that their browser will then render.
In a traditional React app, this process leans heavily on Client-Side Rendering. The server sends a minimal HTML structure with links to JavaScript files. The bulk of the rendering responsibility lies with the user’s browser, which fetches, interprets, and then displays the content. This can lead to a noticeable delay before the user sees the full, interactive page.
Contrast this with Next.js, which emphasizes Server-Side Rendering. Here, the server does most of the heavy lifting. When a user requests a page, the content is “pre-rendered” on the server, resulting in a fully formed HTML page being sent to the browser. This means the user sees the content faster, although not yet fully interactive. The result is a quicker initial page load. Then soon after the initial content is shown, the JavaScript is loaded and executed and the page becomes interactive.
While React can be configured for server-side rendering, Next.js offers this capability out of the box, streamlining the development process.
Winner:
Example
In the comparison below, you can see that Next.js performs the server-side rendering through the getServerSideProps
function that fetches data on the server before rendering the page. Because of this, a pre-rendered page is sent to the user, reducing the initial load time and offering a better experience.
Next.js example: Server-Side Rendering
import React from 'react';
const NextApp = ({ data }) => {
return (
<div>
{data.map((item) => (
<p key={item.id}>{item.name}</p>
))}
</div>
);
};
export async function getServerSideProps() {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return {
props: {
data,
},
};
}
export default NextApp;
React.js Example: Client-Side Rendering
import React, { useEffect, useState } from 'react';
const ReactApp = () => {
const [data, setData] = useState([]);
useEffect(() => {
fetch('https://api.example.com/data')
.then((response) => response.json())
.then((result) => setData(result));
}, []);
return (
<div>
{data.map((item) => (
<p key={item.id}>{item.name}</p>
))}
</div>
);
};
export default ReactApp;
Next.js vs. Static Site Generation Comparison
With server-side rendering, the content is pre-rendered on the server when a user requests a page. This means that each time a user requests a page, the server generates the HTML for that page in real-time and then sends it to the user's browser.
Alternatively, what you could do is pre-render in advance during the build process. This is called Static Site Generation. When a user requests a page, the pre-rendered static HTML file is sent without the need for real-time generation. If your content doesn't change often, static site generation is the way to go since the server can quickly serve the already-generated HTML.
Next.js provides out-of-the-box solutions for both server-side rendering and static site generation. React, on the other hand, requires additional setup and tools to achieve both.
Winner:
Next.js vs. React Code Splitting Comparison
Code splitting is an optimization technique to enhance performance by loading only the code necessary for a specific page.
Example: Next.js Code Splitting
With Next.js, code splitting is seamless. Each page becomes a boundary for code splitting, leading to smaller initial bundle sizes and thus better performance.
// pages/about.js
import React from 'react';
const AboutPage = () => {
// Component code...
};
export default AboutPage;
Example: React.js Code Splitting
In React.js, code splitting requires more manual effort using the lazy
function and Suspense component, potentially leading to more complex setups. React.lazy()
dynamically loads a component and wraps it with Suspense
to handle the loading state. This allows you to load components only when they are needed.
import React, { lazy, Suspense } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
const MyPage = () => {
return (
<div>
<h1>My Page</h1>
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
</div>
);
};
Winner:
Next.js vs. React SEO Comparison
After Googlebot crawls a web page, it renders the HTML and CSS and executes the page’s JavaScript, then finally indexes the content it sees and follows the links from that page to crawl more pages.
Two common problems sites run into during this process:
- Google can’t see their rendered content
- Google can’t follow the links on their page to discover all the other pages on their site
Being client-side rendered, it’s easy for React to run into both of these problems.
Imagine a React-based property search site that loads its property listings via an API call. If the site relies solely on client-side rendering, the initial HTML sent to the browser (and to Googlebot) might only contain placeholders or loading spinners. The actual property listings, which are crucial for SEO, would only appear after the JavaScript fetches the data and updates the page. If Googlebot struggles to execute this JavaScript or if there's a delay in the data fetching, the property listings might not be indexed.
Here’s a real-life example from the warehouse company Goodman. If you load the page in your browser, you’ll see all the property listings:
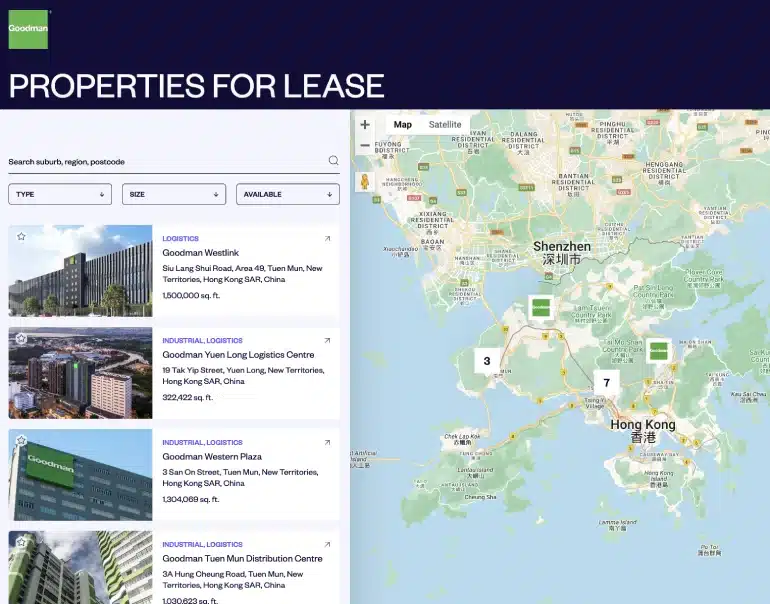
However, from Google’s perspective, using Google’s URL Inspection tool, this is what they see:
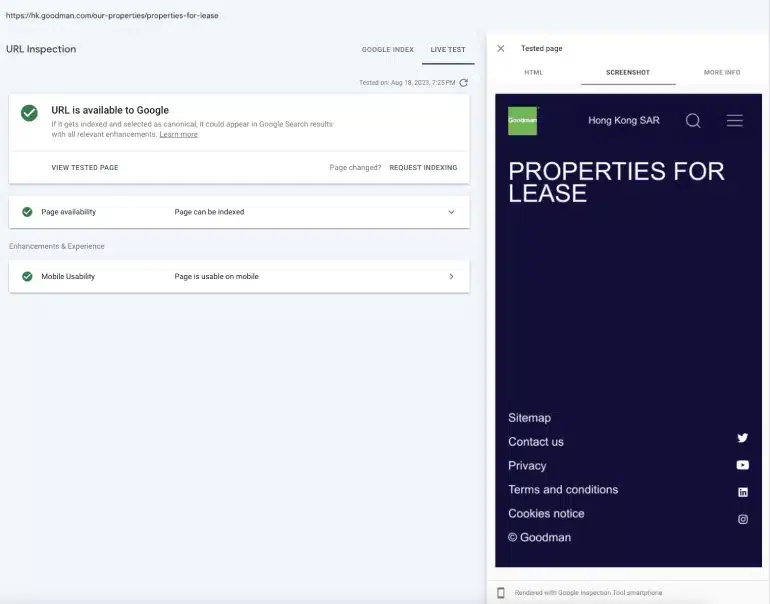
Google rendered and indexed the page before the property listings loaded. Not only is the listing content invisible, but Google doesn’t see the links to individual listing pages either.
To mitigate these SEO challenges, you can implement server-side rendering manually with React, but this requires additional configurations and server setup. Another popular solution is to use static site generators like Gatsby, which pre-renders React apps into static pages, enhancing their SEO friendliness.
On the other hand, Next.js offers server-side rendering by default, making it a go-to choice for developers prioritizing SEO.
Winner:
Next.js is the clear winner
While both Next.js and React stem from the same lineage, in the pursuit of optimal performance, their approaches to rendering make Next.js the clear winner. Next.js should be your go-to if performance is crucial and you want an out-of-the-box solution.
In fact, in a recent update to their "Start a New React Project" documentation, React says, "if you want to build a new app or a new website fully with React, we recommend picking one of the React-powered frameworks popular in the community" and lists Next.js first.
To consistently deliver a superior user experience, don’t forget to track errors
Managing errors and exceptions in your code is challenging. It can make deploying production code an unnerving experience. Being able to track, analyze, and manage errors in real-time can help you to proceed with more confidence. Rollbar automates error monitoring and triaging, making fixing Next.js and React.js errors easier than ever. Try it today!
See our other blog posts comparing frameworks