When React arrived on the scene in 2013, it quickly became the darling of developers by making everything about UI delightful and component-y. Yet as millions embraced React, they also demanded more than just delightful UI. Developers craved snappier performance and better search engine optimization (SEO). That's when Next.js waltzed in, whispered "server-side rendering," and changed the game.
Next.js builds on React's legacy and takes it to new horizons.
But what does this evolution mean for the everyday developer? How does one navigate the nuances between React and Next.js? And perhaps most importantly, when should you choose one over the other?
These are not mere academic questions, but ones that have tangible implications for website performance, user experience, search engine rankings, and overall project success. In this article, we'll unpack the journey from React to Next.js, delve into the unique strengths each brings to the table, and provide insights to guide your next project decision.
What is React?
React is an open-source, front-end JavaScript library that was originally created at Facebook to address challenges that they had in building and maintaining complex user interfaces. Since open-sourcing it, React has grown immensely popular in the developer community thanks to its effective management of state and the creation of reusable UI components. React's virtual DOM speeds up rendering by updating only the user interface elements that have changed, making interactions faster and more fluid.
Example: How to Create a Simple Counter App in React
- Create a React app using the below command:
npx create-react-app counter
- Enter the folder “counter”:
cd counter
- Now just write the below code in the
App.js
file present inside thesrc
folder:import React, { useState } from "react"; import "./App.css"; const App = () => { const [counter, setCounter] = useState(0) const increment = () => { setCounter(counter + 1) } const decrement = () => { setCounter(counter - 1) } return ( <div style={{ display: 'flex', flexDirection: 'column', alignItems: 'center', justifyContent: 'center', fontSize: '300%', position: 'absolute', width: '100%', height: '100%', top: '-15%', }}> React App <div style={{ fontSize: '120%', position: 'relative', top: '10vh', }}> {counter} </div> <div className="buttons"> <button style={{ fontSize: '60%', position: 'relative', top: '20vh', marginRight: '5px', backgroundColor: 'green', borderRadius: '8%', color: 'white', }} onClick={increment}>Increment</button> <button style={{ fontSize: '60%', position: 'relative', top: '20vh', marginLeft: '5px', backgroundColor: 'red', borderRadius: '8%', color: 'white', }} onClick={decrement}>Decrement</button> </div> </div> ) } export default App
- Run the above code using the below command:
npm start
You can see the output at localhost like
localhost:3000
in the browser:Example of simple app created with React.js
What is Next.js?
The Next.js framework was created on top of React by the cloud hosting platform Vercel. You see, a major drawback of websites built with React is that they use client-side rendering (CSR) to navigate between different "pages" or views of the app. Next.js, on the other hand, can support server-side rendering (SSR).
By pre-rendering pages on the server and serving only static HTML files, Next.js offers quicker initial page loads and makes it easier for search engines to crawl and index the site, resulting in better SEO.
Example: How to Create a Simple App in Next.js:
- Create a Next.js app using the below command:
npx create-next-app my-next-project
Select the following options when creating:
- Now go to the folder
my-next-project
cd my-next-project
- Within the
app
app folder go topage.tsx
and type the below code:'use client' import {useState} from 'react' export default function Home() { const [numState,setNumState] = useState<any>(0) const increment = () => { setNumState(numState + 1 ) } const decrement = () =>{ setNumState(numState-1) } return ( <div className='h-screen w-screen flex flex-col justify-center items-center text-[80px]'> <p className='test-[50px]'>My Counter App Next.js</p> <p className='font-bold'>{numState}</p> <div className='flex flex-row gap-20'> <button onClick={increment}>+</button> <button onClick={decrement}>-</button> </div> </div> ) }
- Now just run the app.
npm run dev
You can see the output at localhost like
localhost:3000
in the browser.Example of simple app created by Next.js
What are the use cases of React?
React is an excellent choice for web apps that don’t require search engine crawling (e.g. they’re behind a login wall). With React, you can build:
- Single-page apps (SPAs) with dynamic and interactive user interfaces
- Complex, feature-rich web apps with a focus on reusability and maintainability
- Mobile apps using React Native, which allows developers to use React to build mobile apps for iOS and Android.
- UI components that integrate into larger ecosystems or frameworks
What are the use cases of Next.js?
Any publicly available website that you expect to have lots of new users landing on and that you want Google sending you search traffic, you should use Next.js. Next.js excels in the following scenarios:
- Content-centric websites and blogs, where SEO and initial page load performance are crucial.
- E-commerce websites where SEO, performance, and dynamic content play a significant role.
- Websites with a mix of static and dynamic content, leveraging Next.js's SSR capabilities.
How do React and Next.js differ from each other?
Factor | React | Next.js |
---|---|---|
1. Rendering Approach | React focuses primarily on client-side rendering, in which components’ initial rendering takes place in the browser. The client side is responsible for handling subsequent interactions and updates. | Next.js adds server-side rendering and static site generation capabilities to React applications. Delivering previously rendered content to the client means better page load times and SEO. |
2. File-Based Routing | Developers of React apps frequently need to manually handle routing using tools like React Router. | The arrangement of pages and routes is made simpler by the availability of file-based routing in Next.js, which makes it simple to define routes based on the directory structure of a project. |
3. Data Fetching | In React, data fetching is often done on the client side using libraries like Axios or the Fetch API. | Next.js supports both client-side and server-side data fetching. For server-side data fetching, Next.js provides the getServerSideProps and getStaticProps functions. |
What are the pros and cons of React?
React Pros | React Cons |
---|---|
|
|
What are the pros and cons of Next.js?
Next.js Pros | Next.js Cons |
---|---|
|
|
Which framework is better and when?
The most crucial question is now: which one should you use? Both are strong frameworks and the best one to employ depends largely on your use case. React is the better option when creating complicated user interfaces or interactive single-page applications that aren’t publicly available or, if they are, you don’t care about ranking well in Google for. You have extensive control over rendering and component state, and it offers a flexible and effective way to manage UI components.
When you want to prioritize SEO and initial loading speed for content-focused, public-facing websites such as blogs or e-commerce sites, Next.js, which is built on React, should be your go-to framework.
Both React.js and Next.js are powerful tools for front-end development. To sum them up, React is very useful to build dynamic and interactive user interfaces whereas Next.js is used on top of React to extend its capabilities by giving server side rendering for better performance.
Track, Analyze and Manage Errors With Rollbar
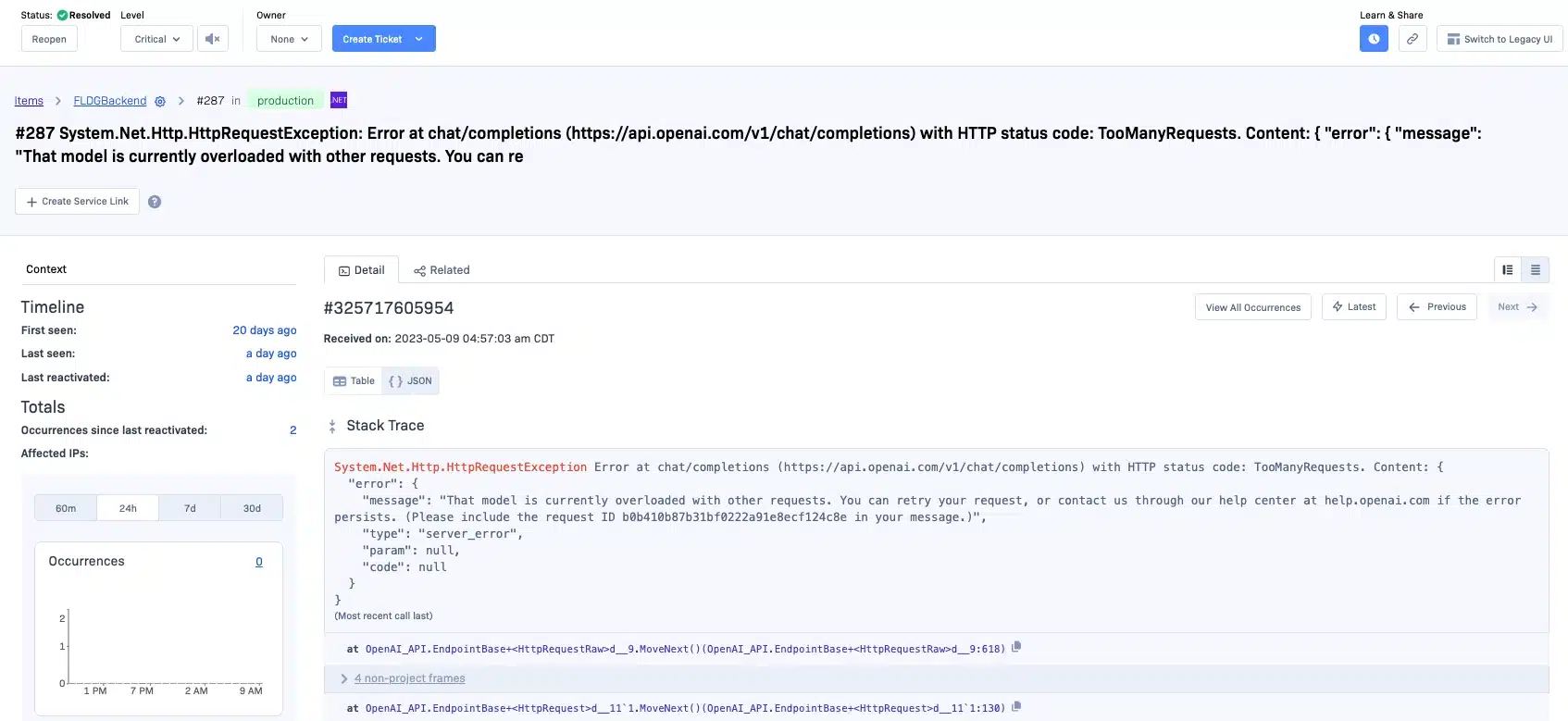
No matter which framework you gravitate towards, ensuring the smooth operation of your app is paramount. Unforeseen errors can be a thorn in your project, disrupting user experience and hindering your app's success. With Rollbar, you can stay a step ahead, tracking and analyzing errors in your React or Next.js apps in real-time. Don't let unexpected issues slow you down. Make the smart move—integrate Rollbar into your workflow today, and ensure that your app is always performing at its peak.
See our other blog posts comparing frameworks: