The choice between Next.js and Remix is not just about picking a framework; it's about embracing a way of thinking that will define the trajectory of your application and your growth as a developer.
Both frameworks cater to modern web development needs, but they differ in their approach to routing, data fetching, and performance optimization.
By understanding the philosophical underpinnings and practical implications of each framework, you can make an informed decision about which path aligns best with our project's goals and your own development philosophy.
1. Routing and Navigation
Next.js uses a file-based routing system where each file in the “pages” directory becomes a route. For example, if you have a file called index.js
in the pages
directory, it will be mapped to the /
route. So to create a new route in Next.js, simply create a new file in the pages
directory.
For example, if you want to create a new route called /about
, you simply create a new file called about.js
in the pages directory:
import React from 'react';
function AboutPage() {
return <div>About Us</div>;
}
export default AboutPage;
Remix, on the other hand, uses a route-centric approach, meaning routes are explicitly mentioned in the configuration file, generally remix.config.js
. This configuration file maps the URLs to route modules. Let’s say you have a Remix project with the following route modules:
src/
├── routes/
│ ├── index.tsx // Represents the root route ("/")
│ ├── about.tsx // Represents the "/about" route
│ └── contact.tsx // Represents the "/contact" route
To explicitly define routes and map URLs to these route modules, you would create or modify your remix.config.js
file like below:
// remix.config.js
module.exports = {
// ...other configuration options...
routes: {
// Define the root route ("/") and specify the entry point
'/': 'src/routes/index.tsx',
// Define the "/about" route and specify the entry point
'/about': 'src/routes/about.tsx',
// Define the "/contact" route and specify the entry point
'/contact': 'src/routes/contact.tsx',
// Add more routes as needed...
},
};
The choice between Next.js and Remix boils down to a preference for simplicity versus explicit configuration. Next.js adopts a file-based routing system, making the creation of new routes as straightforward as adding a new file in the pages
directory. This approach is intuitive and requires minimal setup, making it a great choice for developers who prefer a straightforward, convention-over-configuration routing system.
On the flip side, Remix employs a route-centric approach where routes are explicitly defined in a configuration file. This setup provides a clear overview of all routes and their corresponding entry points in one place, allowing for more control and organization, especially in larger or more complex applications. It's a solid choice for developers who prefer having an explicit routing configuration and don't mind the additional setup for the sake of clarity and control.
The decision hinges on whether you value ease and simplicity or explicit organization and control when managing the routing in your web applications.
2. Data Loading and Fetching
In Next.js, you can use a variety of techniques to fetch data, depending on your needs. For example, you can use:
getServerSideProps
to fetch data on the server and render it on the server. It is a good option for dynamic data that needs to be updated frequently.getStaticProps
to fetch data on the server and render it statically. It is a good option for static data that doesn't change often.getInitialProps
to fetch data on the server and pass it to the client. It is a good option for data that needs to be fetched on the server but can be updated on the client.
Client-side data fetching in Next.js
is typically done with standard React useEffect
or fetch calls.
For instance, using getStaticProps
for server-side rendering:
// pages/index.js
import React from 'react';
function HomePage({ message }) {
return <div>{message}</div>;
}
export async function getStaticProps() {
const response = await fetch('https://api.example/data');
const data = await response.json();
return {
props: {
message: data.message,
},
};
}
export default HomePage;
In Remix, the concept of loaders is used to fetch data for a route. These can be defined for both server-side and client-side data fetching. This approach provides a unified way to manage data loading across our application.
// routes/about.js
import { json, LoaderFunction } from '@remix-run/node';
export let loader: LoaderFunction = async ({ request }) => {
const response = await fetch('https://api.example.com/about');
const data = await response.json();
return json({ message: data.message });
};
export default function About({ message }) {
return <div>{message}</div>;
}
For someone new to both frameworks, learning three different data fetching methods in Next.js is a steeper learning curve compared to learning the single loader concept in Remix.
The other thing to consider is since data fetching methods in Next.js are placed alongside the page components, this can lead to large files if the data fetching logic is complex. Remix encourages placing loaders in a separate file or alongside the route module, which can lead to better organization, especially in applications with complex data fetching logic.
3. Performance Optimization
While both frameworks are engineered for high performance, Remix offers better dynamic content delivery, faster build times, and a more straightforward approach to handling data. On the other hand, if your project has a strong emphasis on delivering static content efficiently, and you’re already familiar with Next.js, there’s no reason to move away from it.
Remix’s edge is in serving dynamic content. It's designed to deliver fast user experiences even on slow networks, automatically handling errors, interruptions, and race conditions which Next.js doesn't handle out of the box.
Next.js encourages the use of client-side JavaScript for serving dynamic content and requires it for data mutations. On the contrary, Remix doesn't rely on client-side JavaScript for these tasks, leading to faster interactions and less data over-fetching.
As projects scale, Next.js build times increase linearly with your data, which could slow down the development process. Remix, though, boasts nearly instant build times that are decoupled from data, making it a more scalable choice for larger projects.
Choose Next.js for its Mature Ecosystem, Remix for Handling Dynamic Data Efficiently
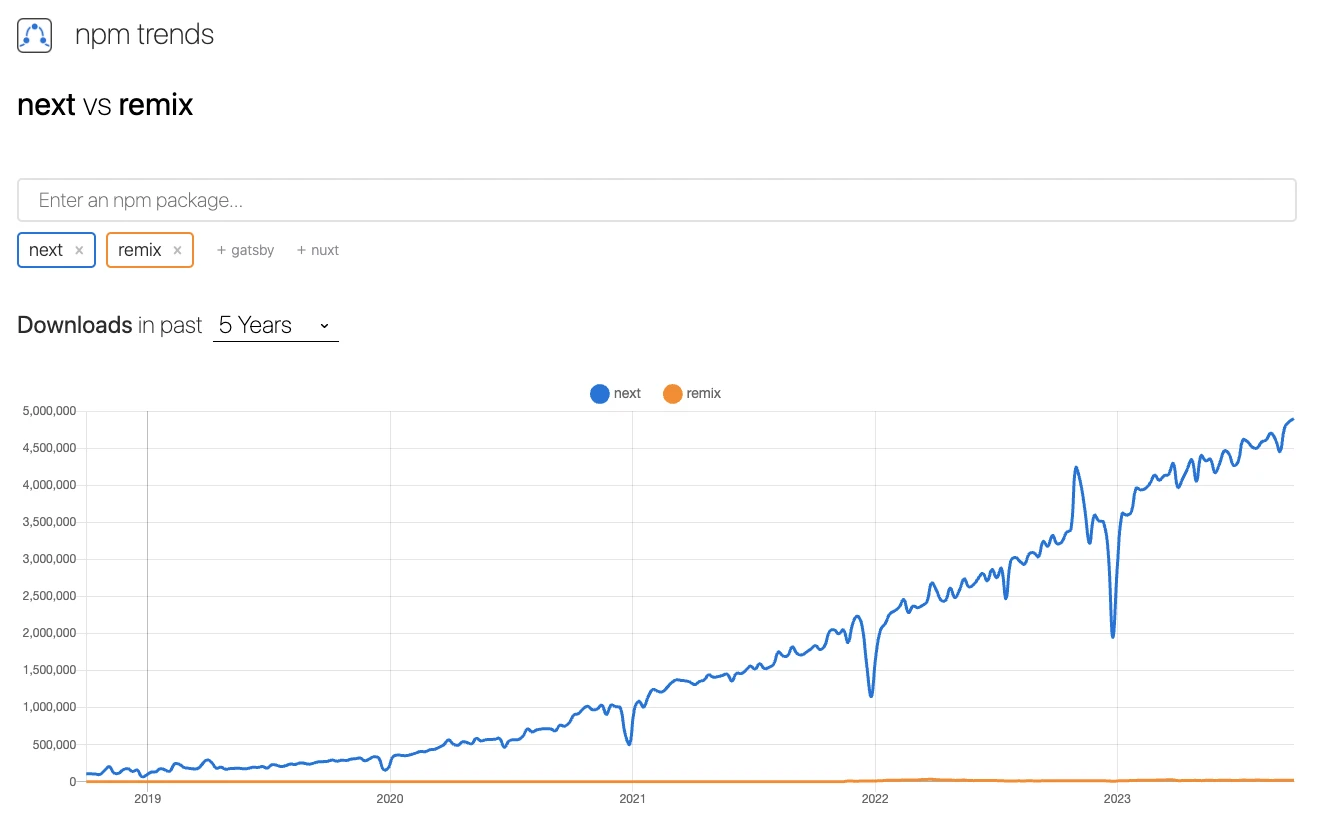
Next.js is a well-established framework with a larger community and ecosystem than Remix, a significant advantage when looking for solutions to problems, plugins, or integrations.
That said, Remix was clearly designed to improve developer productivity with its route-centric development and fine-grained control over data loading and navigation. It provides a performance edge especially in applications with complex data requirements.
For a project that serves a vast amount of static content like an e-commerce site’s product pages, category pages, and blog posts, Next.js would be a wise choice due to its strong support for static site generation and many integrations.
However, if you’re building something like a real-time dashboard that must handle dynamic data efficiently, provide fast user experiences even on slow networks, and allow for flexible routing to manage the complex navigation requirements of the dashboard, Remix would be a more fitting choice. The route-centric development and unified data fetching method provided by Remix would allow for complex routing scenarios and efficient handling of dynamic data.
In the end, both frameworks are robust and capable, and your choice will ultimately depend on your project's long-term vision and the development experience you seek to achieve.
Track, Analyze and Manage Errors With Rollbar
No matter which framework you gravitate towards, ensuring the smooth operation of your app is paramount. Unforeseen errors can be a thorn in your project, disrupting user experience and hindering your app's success. With Rollbar, you can stay a step ahead, tracking and analyzing errors in your Next.js or Remix apps in real-time. Don't let unexpected issues slow you down. Make the smart move—integrate Rollbar into your workflow today, and ensure that your app is always performing at its peak.
See our other blog post comparing frameworks